Box Layouts¶
Box layouts are basic building blocks for dynamic widget layouting.
Box layouts either stack widgets vertically (Tui::ZVBoxLayout
) or place them side by side horizontally
(Tui::ZHBoxLayout
).
Additionally they allow to add automatic spacing between widgets, manual spacing between widgets and stretch spacing that acts a bit like a spring.
Stretch¶

Horizontal box layout with stretch before 2 buttons¶
Example Code
Tui::ZHBoxLayout *layout = new Tui::ZHBoxLayout();
layout->setSpacing(1);
widget->setLayout(layout);
layout->addStretch();
Tui::ZButton *item1 = new Tui::ZButton(Tui::withMarkup, "Button1", widget);
layout->addWidget(item1);
Tui::ZButton *item2 = new Tui::ZButton(Tui::withMarkup, "Button2", widget);
layout->addWidget(item2);

Horizontal box layout with stretch between 2 buttons¶
Example Code
Tui::ZHBoxLayout *layout = new Tui::ZHBoxLayout();
layout->setSpacing(1);
widget->setLayout(layout);
Tui::ZButton *item1 = new Tui::ZButton(Tui::withMarkup, "Button1", widget);
layout->addWidget(item1);
layout->addStretch();
Tui::ZButton *item2 = new Tui::ZButton(Tui::withMarkup, "Button2", widget);
layout->addWidget(item2);
Stretch elements allow creating space between items in the layout that expands to fill the available width.
They take space at the same priority as widgets using a expanding size policy.
Typical usage is placing a stretch element as left most element to align the following widgets to the right.
Placing one stretch element between widgets creates a space in the middle and pushes the widgets towards the edge of the layout.
Nested¶
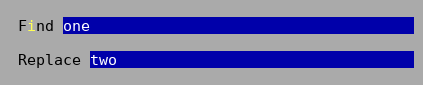
Horizontal box layout nested within vertial box layout.¶
Example Code
Tui::ZVBoxLayout *vbox = new Tui::ZVBoxLayout();
vbox->setSpacing(1);
widget->setLayout(vbox);
Tui::ZHBoxLayout *hbox1 = new Tui::ZHBoxLayout();
hbox1->setSpacing(1);
Tui::ZLabel *labelFind = new Tui::ZLabel(Tui::withMarkup, "F<m>i</m>nd", widget);
hbox1->addWidget(labelFind);
Tui::ZInputBox *searchText = new Tui::ZInputBox(widget);
labelFind->setBuddy(searchText);
searchText->setText("one");
hbox1->addWidget(searchText);
vbox->add(hbox1);
Tui::ZHBoxLayout *hbox2 = new Tui::ZHBoxLayout();
hbox2->setSpacing(1);
Tui::ZLabel *labelReplace = new Tui::ZLabel(Tui::withMarkup, "Replace", widget);
hbox2->addWidget(labelReplace);
Tui::ZInputBox *replaceText = new Tui::ZInputBox(widget);
labelReplace->setBuddy(replaceText);
replaceText->setText("two");
hbox2->addWidget(replaceText);
vbox->add(hbox2);
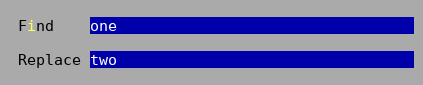
Vertial box layout nested within horizontal box layout.¶
Example Code
Tui::ZHBoxLayout *hbox = new Tui::ZHBoxLayout();
hbox->setSpacing(1);
widget->setLayout(hbox);
Tui::ZVBoxLayout *vbox1 = new Tui::ZVBoxLayout();
vbox1->setSpacing(1);
Tui::ZLabel *labelFind = new Tui::ZLabel(Tui::withMarkup, "F<m>i</m>nd", widget);
vbox1->addWidget(labelFind);
Tui::ZLabel *labelReplace = new Tui::ZLabel(Tui::withMarkup, "Replace", widget);
vbox1->addWidget(labelReplace);
hbox->add(vbox1);
Tui::ZVBoxLayout *vbox2 = new Tui::ZVBoxLayout();
vbox2->setSpacing(1);
Tui::ZInputBox *searchText = new Tui::ZInputBox(widget);
labelFind->setBuddy(searchText);
searchText->setText("one");
vbox2->addWidget(searchText);
Tui::ZInputBox *replaceText = new Tui::ZInputBox(widget);
labelReplace->setBuddy(replaceText);
replaceText->setText("two");
vbox2->addWidget(replaceText);
hbox->add(vbox2);
Nesting layouts allows for more complex arrangements of widgets. It is important to consider in which order the items are nested, because nested box layouts do not create a grid, but each layout’s items are layouted individually.
ZVBoxLayout¶
-
class Tui::ZHBoxLayout : public Tui::ZLayout¶
This layout arranges items horizontally. Items are placed from left to right.
-
int spacing() const¶
-
void setSpacing(int sp)¶
The
spacing
determines the amount of blank cells betweeen layouted items.It does not apply if one of the adjacent items itself is a spacer (according to
bool Tui::ZLayoutItem::isSpacer() const
).
-
void addSpacing(int size)¶
Add manual spacing of
size
blank cells. Spacing set via :cpp:func::void setSpacing(int sp)` does not apply for this spacing.
-
int spacing() const¶
ZHBoxLayout¶
-
class Tui::ZVBoxLayout : public Tui::ZLayout¶
This layout arranges items vertically. Items are placed from top to bottom.
-
int spacing() const¶
-
void setSpacing(int sp)¶
The
spacing
determines the amount of blank cells betweeen layouted items.It does not apply if one of the adjacent items itself is a spacer (according to
bool Tui::ZLayoutItem::isSpacer() const
).
-
void addSpacing(int size)¶
Add manual spacing of
size
blank cells. Spacing set via :cpp:func::void setSpacing(int sp) does not apply for this spacing.
-
void addStretch()¶
Add a stretch item that acts like a spring in the layout and takes additional space with the same priority as :cpp:enumerator::Expanding <Tui::SizePolicy::Expanding> items.
-
int spacing() const¶